Simpler and Better Flutter State Management with Cubit
Learn how to use Cubit to cleanly organise our Flutter codebase to be more readable, scalable, and testable.
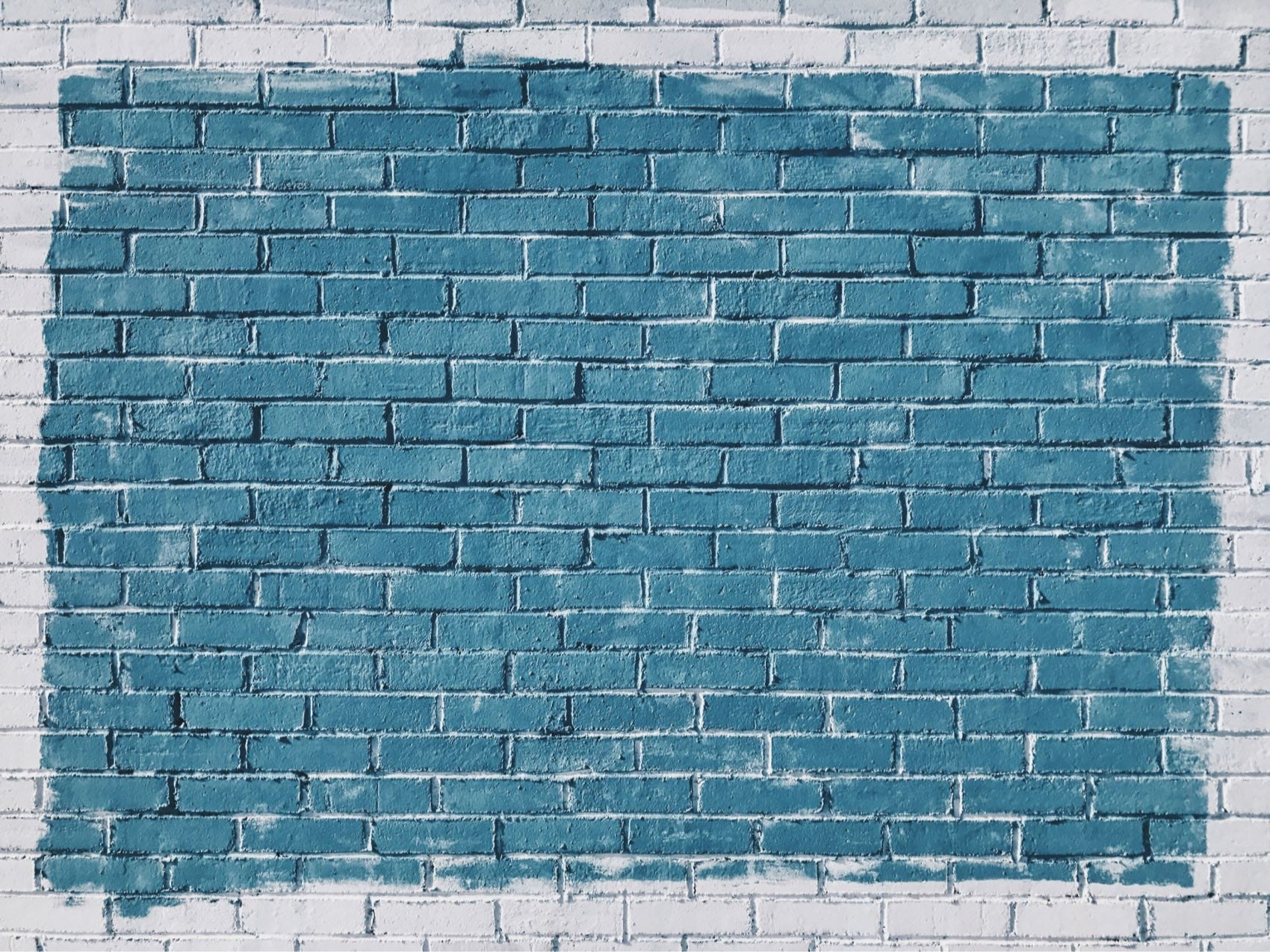
Learn how to use Cubit to cleanly organise our Flutter codebase to be more readable, scalable, and testable.